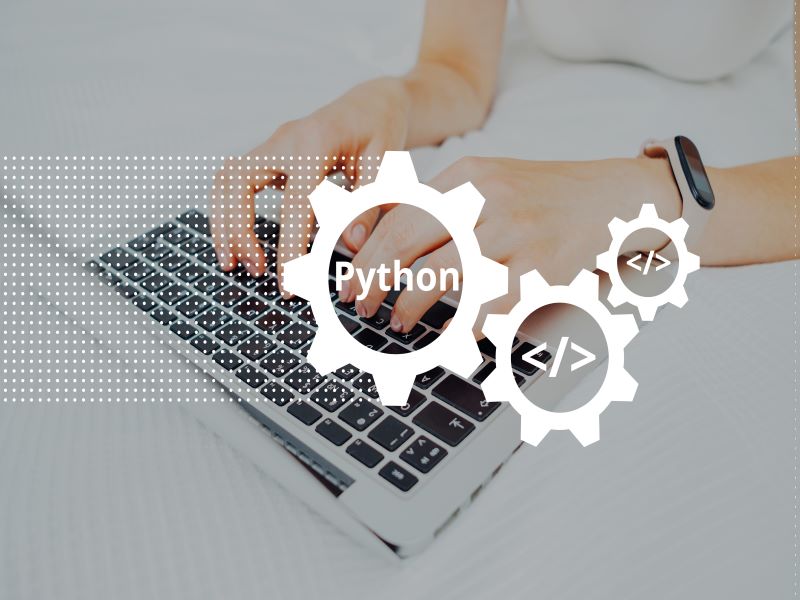
Python Programming for Beginners
Aydoğan EMSİZ
Software Engineer
Aktif Mühendislik
Summary — What is the Python programming language for beginners? Why Python? What can be done with Python? This is an article which we find answers to the questions that mantioned above, review the sample Python codes, and include recommendations for advanced Python programming.
I. WHAT IS PYTHON?
Python, is an object-oriented, interpretive, modular and interactive high-level programming language. [1]
Python was developed by Guido van Rossum in 1990 in Amsterdam, and is currently being developed by the efforts of volunteers gathered around the Python Software Foundation.
Simple syntax based on indentations makes it easier to learn and understand the language for beginners.
It can work on many platforms. (Unix, Linux, Mac, Windows, Amiga, Symbian and more.)
II. WHY PYTHON?
Why use Python when there are dozens of programming languages to use? Let’s give the best answer to the question with the advantages listed below Python.
- Have an easy-to-read, readable, and understandable syntax.
- Can work on almost all platforms.
- Suitable for object-oriented programming.
- Having strong libraries in many areas. (SciPy, NumPy, Matplotlib, SageMath, Deepy, Caffe, OpenCV, Zerynth, Orange, PyData)
- Use and support by large firms. (Google, Wikipedia, Yahoo, CERN, NASA, Reddit)
- Continuous increase in popularity and number of users.
We can detail these advantages with examples and statistics.
First, compare the C # and Python programming languages and see the difference between them.
For example, let’s try to get “Hello World!” printouts in both languages.
C# Syntax
using System; using System.Collections.Generic; using System.Text; namespace ConsoleApplication1 { class Program { static void Main(string[] args) { Console.WriteLine("Hello World!"); } } }
Python Syntax
print("Hello World!")
We see the difference in syntax and the advantage of Python.
Now, let’s take a look at the PYPL PopularitY of Programming Language list, which was created using Google Trends raw data based on learning programming languages in Google searches. [2]
Worldwide, the comparison with a year ago, in December 2018:
The statistics below show that Python is the world’s most popular language as of December 2018. Python grew the most in the last 5 years (15.4%) and PHP lost the most (-6.3%)
Yes, that’s because of all these advantages Python!
III. WHAT CAN BE DONE WITH PYTHON?
Python is a programming language used in many areas. With Python, applications can be developed in various fields such as web applications, desktop applications, game development, mobile application, network programming, data mining, data analysis, machine learning, artificial intelligence applications, scientific computing applications, Internet of Things (IoT).
There are very large libraries available for the fields mentioned above and more.
Sample Projects Enhanced with Python:
Reddit, Django, Portage, Sage, GNU Mailman, Maya, Blender, Inkscape
For more you can view the article “30 Amazing Python Projects for the Past Year (v.2018)”
IV. PYTHON PROGRAMMING FOR BEGINNERS
A. Python Installation
Before starting programming with Python, we need to install Python on our computer.
We can download the appropriate Python version from our Python Software Foundation official site, https://www.python.org/downloads/
Detailed installation notes for Windows, OS X, Linux and other operating systems are available at https://tutorial.djangogirls.org/en/python_installation/
We need an IDE or editor to write Python codes after installation.
You can choose one of the most used editors below:
Jupyter, PyCharm, Spyder, Visual Studio Code, Sublime Text, Eclipse + PyDev, Atom, Visual Studio
We need to know Python data types, type conversions and operators before starting to code.[3][4]
B. Python Data Types
Data Type | Description | Sample |
---|---|---|
Integer – int | Integer data type. 32 bit. Defines the exact numbers between ±2147483647. | 25 |
Long integer – long int | Is a wider range of integer data types. 64 bit. | 9876543210 |
Float – float | Floating point data type. 64 bit double precision. Used to define decimals. | 3.14 |
Complex – complex | Complex number data type. | a+bj |
Boolean – bool | Logical data type. | a+bj |
String – string | Used to display character strings. Double quotes, single quotes, or inside quotes are shown. | ‘Merhaba’, “””Dünya”””, “Aydoğan’ın” |
List – list | Used to identify different objects. The square brackets are shown in []. Can host different data types. List content can be changed. | [3, [4, ‘four’], 4.5, “Aydoğan”] |
Tuple – tuple | It is similar to the lists. Normal brackets are shown in (). List contents cannot be changed. | (1, ‘test’, 4, ‘U’) , tuple(‘test’) |
Dictionary – dictionary | Dictionary data type. It is defined within the spring brackets {}. | {‘food’: ‘adana kebap’, ‘drink’: ‘acılı şalgam’} |
In Python variable definitions, there is no need to specify data type as in other languages. Python automatically determines the variable’s data type as soon as an assignment is made.
For example, when a = 2, the data type of ‘a’ is set to integer.
C. Python Type Conversions
Function | Description |
---|---|
int(x [,base]) | Converts the variable x to the specified base type. |
long(x [,base] ) | Converts the variable x to the specified base type. |
float(x) | Converts the variable x to decimal. |
complex(real [,imag]) | Converts to complex number. |
str(x) | Converts the x variable to a string type. |
repr(x) | Returns a string containing a printable representation of an object a. |
eval(str) | Returns a string containing a printable representation of an ‘a’ object. |
tuple(x) | Converts the variable x to tuple. The contents cannot be changed. |
list(x) | Converts the x variable to the list type. |
set(x) | Converts the x object to the setting object. |
dict(x) | Converts the x object to the dictionary object. x (key, value). |
frozenset(x) | Converts the x object to the frozenset object. |
chr(x) | Converts an integer to a character. |
unichr(x) | Converts an integer to unicode. |
ord(x) | Converts an odd character to an integer. |
hex(x) | Converts an integer to a hexadecimal value. |
oct(x) | Converts an integer to an octal value. |
D. Python Operators:
Operator | Description | Sample |
---|---|---|
+ | Collects the values on the right and left of the operator. | x = y + z |
– | Subtracting values on the right and left of the operator. | x = y – z |
* | It multiplies the values on the right and left of the operator. | x = y * z |
/ | Divides y by z. | x = y / z |
% | Returns the remainder of the Y value divided by the Z value. | x = y % z |
** | y is the multiplication of the time z. | x = y ** z |
// | Rounds the result of the division to an integer. | x = y // z |
Now we’re ready to start sample codes.
E. Python Sample Codes[5]
#1: Python code that prints “Hello World!” print("Hello World!")
>>> Output:
Hello World!
#2: Comment and description line example a=2 #One-line comments are written with the “#” sign. """ Multi-line comments and explanations start with 3 double or single quotes and end with the same mark. Codes in comment lines are not processed.a = 10 """ print(a)
>>> Output:
2
#3: Example of Python that prints the name of the user and prints “Hello userName” userName = input('Enter Your Name : ') print("Hello " + userName)
>>> Output:
Enter Your Name : Aydoğan
Hello Aydoğan
#4: Example of Python that compiles the 2 numbers entered number1 = input('Number 1 : ') number2 = input('Number 2 : ') total = float(number1) + float(number2) print("Total : {0} ".format(total))
>>> Output:
Number 1 : 4
Number 2 : 12.5
Total :16.5
#5: An example of a Python that averages 2 numbers entered number1 = input('Number 1 : ') number2 = input('Number 2 : ') avgNumbers = (int(number1) + int(number2)) / 2 print("Average : {0} ".format(avgNumbers))
>>> Output:
Number 1 : 7
Number 2 : 38
Average : 22.5
#6: Example of Python that found whether the number entered is prime or not count = 0 number = input('Number: ') for i in range(2, int(number)): if(int(number) % i == 0): count += 1 break if(count != 0): print("Number is not prime") else: print("Number is prime")
>>> Output:
Number: 7
Number is prime
#7: Example of Python calculating the average of the entered midterm and final grade midterm = input('Midterm Point : ') final = input('Final Point : ') avgPoint = (float(midterm) * 0.3) + (float(final) * 0.7) print("Average :{0} ".format(avgPoint))
>>> Output:
Midterm Point : 35
Final Point : 75
Average :63.0
#8: Python example averaging 3 entered exams e1 = input('Exam 1 : ') e2 = input('Exam 2 : ') e3 = input('Exam 3 : ') avgExam = (float(e1) + float(e2) + float(e3)) / 3 print("Avg Exams :{0} ".format(avgExam))
>>> Output:
Exam 1 : 40
Exam 2 : 55
Exam 3 : 70
Average :55.0
#9: An example of a Python example that shows the student's grade passing status (PASSED - FAILED) avgPoint = input('Enter your average : ') if(int(avgPoint) >= 50): print("PASSED") else: print("FAILED")
>>> Output:
Enter your average : 53
PASSED
#10: An example of a Python that finds whether the entered number is odd or even. number = input('Number : ') if(int(number) % 2 == 0): print("Number is even") else: print("Number is odd")
>>> Output:
Number : 33
Number is odd
#11: An example of Python that finds the number entered is positive, negative, or zero number = input('Number : ') if(int(number) < 0): print("Number is negative") elif(int(number) > 0): print("Number is positive") else: print("Number is zero")
>>> Output:
Number : -42
Number is negative
#12: An example of Python that indicates whether the person whose age is entered can get a driver's license age = input('Enter your age : ') if(int(age) < 18): print("Your age is not eligible for driving license") else: print("Your age is eligible for driving license")
>>> Output: Enter your age : 17
Your age is not eligible for driving license
#13: Python example listing numbers from 1 to 100 on the screen. for i in range(1, 101): print(i)
>>> Output:
1
2
3…
…100
#14: An example of Python that lists the even numbers from 1 to 100. for i in range(1, 101): if i % 2 == 0: print(i)
>>> Output:
2
4
6…
…100
#15: An example of Python that lists the odd numbers from 1 to 100. for i in range(1, 101): if i % 2 != 0: print(i)
>>> Output:
1
3
5…
…99
#16: An example of a python that finds the area of the rectangle and its surroundings shortEdge = input('Short Edge : ') longEdge = input('Long Edge : ') area = int(shortEdge) * int(longEdge) surroundings = 2 * (int(shortEdge) + int(longEdge)) print("Area : {0}".format(area)) print("Surroundings : {0}".format(surroundings))
>>> Output:
Short Edge : 6
Long Edge : 10
Area : 60
Surroundings : 32
#17: Example of Python showing the sum of numbers between two numbers entered by the user. total = 0 number1 = input('Number 1: ') number2 = input('Number 2: ') for i in range(int(number1) + 1, int(number2)): total += i print("Sum of numbers between {0} and {1}: {2}".format(number1, number2, total))
>>> Output:
Number 1: 12
Number 2: 24
Sum of numbers between 12 and 24: 198
#18: The example of Python, which calculates the salary of the worker who entered the salary and the raise rate on the screen newSalary = 0 oldSalary = input("Enter old salary : ") raiseRatio = input("Enter raise ratio(%) : ") newSalary = int(oldSalary) + (int(oldSalary) * int(raiseRatio) / 100) print("New Salary :", newSalary)
>>> Output:
Enter old salary : 2000
Enter raise ratio(%) : 30
New salary : 2600.0
#19: Example of Python using the function to calculate the radius of the circle entered by radius def calcArea(radius): area = float(radius) * float(radius) * 3.14 print ("Area :", area) return alan r = input("Enter radius :") calcArea(r)
>>> Output:
Enter radius :5
Area: 78.5
#20: Example of number guessing game with Python. from random import randint rand = randint(1, 100) count = 0 while True: count += 1 number = int(input("Enter value from 1 to 100 (0 exit):")) if(number == 0): print("You're exit of the game") break elif number < rand: print("Enter a Higher Number.") continue elif number > rand: print("Enter a Lower Number.") continue else: print("Randomly selected number {0}!".format(rand)) print("Count of Estimates {0}".format(count))
>>> Output:
Enter value from 1 to 100 (0 exit): 6
Enter a Higher Number.
Enter value from 1 to 100 (0 exit): 15
Enter a Higher Number.
Enter value from 1 to 100 (0 exit): 95
Enter a Lower Number.
Enter value from 1 to 100 (0 exit): 92
Enter a Higher Number.
Enter value from 1 to 100 (0 exit): 93
Randomly selected number 93!
Count of Estimates 5
Enter value from 1 to 100 (0 exit):0
You’re exit of the game
#21: An example of a Python instance that checks if a character is specified in a string. The control is done in the function. def checkCharacter(str): count = 0 for ch in str: if ch == 'ğ': count = count + 1 if count == 1: return True else: return False strValue = input('Enter text:') if(checkCharacter(strValue) == True): print('The character ğ is in text') else: print('The character ğ is not in text')
>>> Output:
Enter Text : aydoğan
The character ğ is in text
For more examples, visit this page:
http://nbviewer.jupyter.org/github/mustafamuratcoskun/Sifirdan-Ileri-Seviyeye-Python-Programlama/tree/master/
V. ADVANCED PROGRAMMİNG WİTH PYTHON
I also recommend Udemy online course for Advance Python Programming like Machine Learning Pyth, which includes topics such as Map Management, Data Analysis (Numpy and Pandas), Machine Learning, Web Programming (Django).
In addition, this website helps to learn Advanced Data Structures and Objects, Advanced Features of Functions and Decorators, Iterators and Generators, Advanced Modules, PyQT GUI Development
Finally, you can visit this page for free Python learning:
https://www.youtube.com/playlist?list=PLqG356ExoxZWbfIhu2C7b0dKIGHKeGKcM
REFERENCES
[2] https://medium.com/yaz%C4%B1l%C4%B1ma-dair/pyhton-programlama-dili-neden-giderek-pop%C3%BClaritesini-artt%C4%B1r%C4%B1yor-ab14b1da39c6
[3] https://orulluogluyusuf.wordpress.com/2016/07/08/pythonda-degiskenler-veri-tipleri/
[4] https://baykoch.github.io/blog/python/python3-veri-tipleri-say%C4%B1lar/
[5] https://www.yazilimkodlama.com/programlama/python-ornekleri/